Single Linked List
First of all, what is Linked List? Linked List is pretty similar to array, but Linked List is a linear data structure. Unlike arrays, linked list elements are not stored at a contiguous location; the elements are linked using pointers.
///Then, what is special about Linked Lists? Are they are just similar to Array?///
Even though array and linked lists are pretty similar, but there is some differences between arrays and linked lists. Here is the difference between array and linked list.
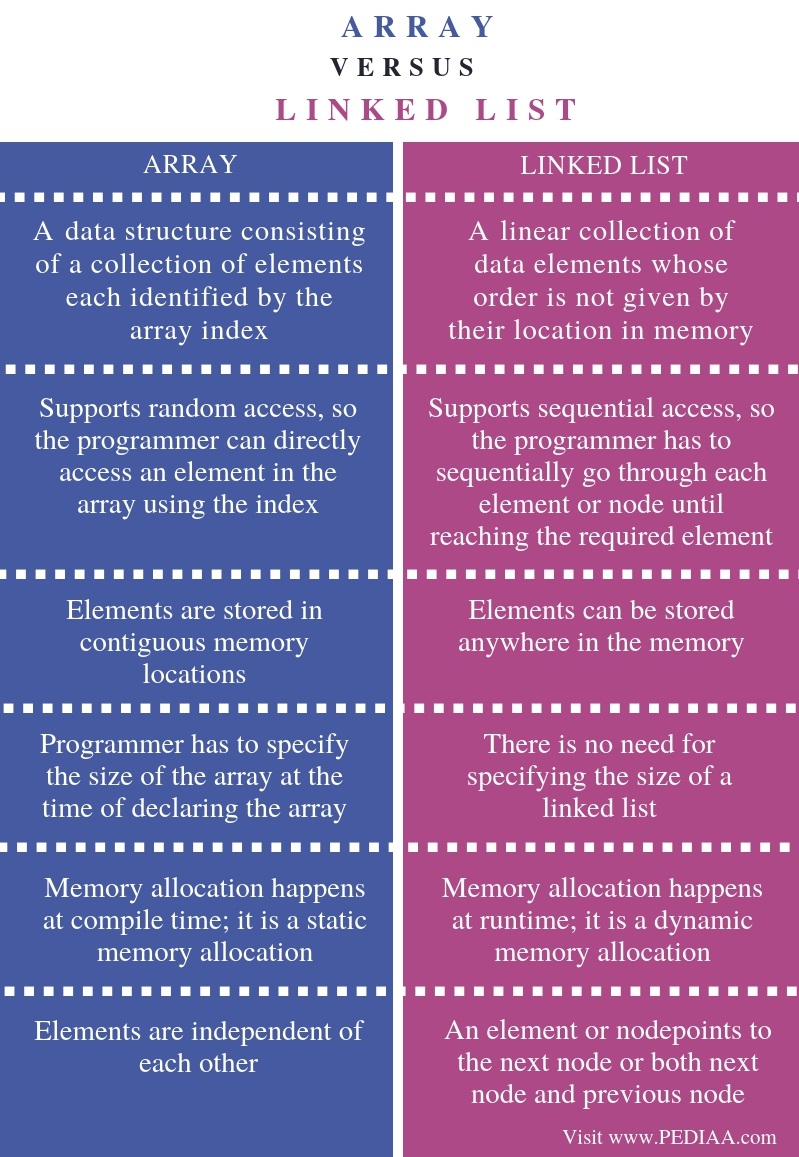
What is Single Linked List? Single Linked List is a type of Linked Lists that only have a single pointer variable that points to the next element that will leads to the tail of Linked List, and the tail will points NULL.
here is the illustration of Single Linked List :
P. S. : Head is the first element of Linked List and Tail is the last element of Linked List
example of 'struct' code :
struct Scholar{
char name[25];
int age;
struct Scholar *next;
}*head,*tail;
char name[25];
int age;
struct Scholar *next;
}*head,*tail;
Memory Allocation in Linked List
In C or C++ programming language, we could use 'malloc' for allocating the memory, and for delete the allocation memory we could use 'free'. The 'free' function usage is for clearing the memory allocation, but not the value of the element in Linked List.
Here is an example of malloc usage :
Insertion and Deletion of Nodes in Single Linked List
Insertion (also known as push) and deletion (also known as pop) is executed in the head, tail or even in the middle of linked list
- Insertion on Head of Linked List
This function is used for inserting or adding the element at the head of Linked List.
Here is the example of code for Insertion at Head :
Here is the example of code for Insertion at Head :
/// Insertion at Head of Linked List in C///
- Insertion on Tail of Linked List
This function is used for inserting or adding the element at the tail of Linked List.
Here is the example of code for Insertion at Tail :
Here is the example of code for Insertion at Tail :
/// Insertion at Tail of Linked List in C///
- Insertion at Middle of Linked List
This function is used for inserting or adding the element at the middle of Linked List.
Here is the example of code for Insertion at Middle :
Here is the example of code for Insertion at Middle :
/// Insertion at Middle of Linked List in C///
- Deletion at Head of Linked List
This function is used for deleting the element at the head of Linked List.
Here is the example of code for Deletion at Head :
Here is the example of code for Deletion at Head :
/// Deletion at Head of Linked List in C///
- Deletion at Middle of Linked List
This function is used for deleting the element at the middle of Linked List.
Here is the example of code for Deletion at Middle :
Here is the example of code for Deletion at Middle :
/// Deletion at Middle of Linked List in C///
- Deletion at Tail of Linked List
This function is used for deleting the element at the tail of Linked List.
Here is the example of code for Deletion at Tail :
Here is the example of code for Deletion at Tail :
/// Deletion at Tail of Linked List in C///
Source :
3. https://pediaa.com/wp-content/uploads/2018/12/Difference-Between-Array-and-Linked-List- Comparison-Summary.jpg
5. http://albertius-christopher-nathan.blogspot.com/2020/03/linked-list-halo-semuanya-topik-kita.html
Komentar
Posting Komentar